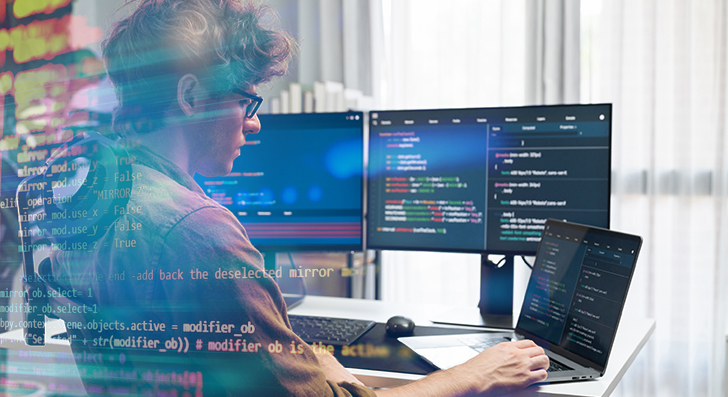
Scalability suggests your software can cope with progress—much more users, extra facts, plus much more targeted traffic—with out breaking. As a developer, making with scalability in mind will save time and anxiety later. Right here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on later—it ought to be part of your respective strategy from the start. Numerous apps fail if they increase fast mainly because the original layout can’t handle the extra load. To be a developer, you should Assume early about how your procedure will behave under pressure.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever everything is tightly linked. As a substitute, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or assistance can scale By itself with no influencing The complete technique.
Also, consider your database from working day a person. Will it require to manage 1,000,000 buyers or simply a hundred? Select the appropriate style—relational or NoSQL—according to how your knowledge will improve. Plan for sharding, indexing, and backups early, even if you don’t want them still.
One more vital point is to prevent hardcoding assumptions. Don’t compose code that only performs underneath present-day conditions. Consider what would happen In case your user base doubled tomorrow. Would your app crash? Would the databases decelerate?
Use structure styles that support scaling, like information queues or party-driven methods. These assist your app deal with more requests without obtaining overloaded.
Whenever you build with scalability in your mind, you're not just getting ready for achievement—you are decreasing foreseeable future head aches. A effectively-planned system is less complicated to maintain, adapt, and grow. It’s better to arrange early than to rebuild afterwards.
Use the best Database
Deciding on the suitable database is actually a important Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the wrong you can slow you down or even bring about failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows in a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. They're strong with associations, transactions, and consistency. Additionally they support scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and data.
If your knowledge is more versatile—like user exercise logs, product or service catalogs, or paperwork—think about a NoSQL solution like MongoDB, Cassandra, or DynamoDB. NoSQL databases are much better at managing massive volumes of unstructured or semi-structured data and might scale horizontally extra very easily.
Also, take into consideration your go through and produce patterns. Do you think you're doing a great deal of reads with less writes? Use caching and read replicas. Do you think you're managing a hefty write load? Take a look at databases that will cope with high compose throughput, as well as function-based information storage devices like Apache Kafka (for non permanent information streams).
It’s also sensible to Imagine ahead. You may not need to have advanced scaling options now, but choosing a database that supports them indicates you gained’t need to have to change later on.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your access patterns. And often keep an eye on database effectiveness when you mature.
In brief, the proper database depends upon your app’s framework, velocity needs, and how you assume it to mature. Take time to pick wisely—it’ll preserve loads of difficulty later on.
Enhance Code and Queries
Quickly code is vital to scalability. As your application grows, just about every smaller hold off provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your process. That’s why it’s essential to Make productive logic from the start.
Start by crafting cleanse, very simple code. Prevent repeating logic and take away everything unneeded. Don’t pick the most advanced Option if an easy 1 works. Keep the features limited, targeted, and easy to check. Use profiling resources to locate bottlenecks—areas exactly where your code takes way too extended to operate or employs an excessive amount of memory.
Upcoming, have a look at your database queries. These normally slow matters down much more than the code by itself. Be certain Every single question only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find particular fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular throughout huge tables.
When you discover precisely the same data getting asked for again and again, use caching. Retailer the results temporarily employing tools like Redis or Memcached which means you don’t should repeat highly-priced operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and helps make your application extra efficient.
Remember to examination with significant datasets. Code and queries that work good with one hundred documents could possibly crash once they have to deal with one million.
In brief, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when required. These methods assistance your application remain clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to handle much more consumers and a lot more website traffic. If all the things goes through 1 server, it'll rapidly become a bottleneck. That’s exactly where load balancing and caching come in. These two tools aid maintain your app fast, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. In place of one particular server undertaking each of the function, the load balancer routes users to different servers based on availability. This implies no solitary server gets overloaded. If a person server goes down, the load balancer can mail visitors to the Other individuals. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused rapidly. When users request the exact same data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. It is possible to serve it through the cache.
There are two prevalent sorts of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static documents near the consumer.
Caching reduces databases load, enhances pace, and can make your app additional effective.
Use caching for things which don’t change generally. And often be certain your cache is up to date when facts does change.
Briefly, load balancing and caching are simple but strong applications. With each other, they help your app tackle much more end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that permit your app develop simply. That’s wherever cloud platforms and containers are available. read more They give you flexibility, minimize setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon Website Services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really need to acquire hardware or guess foreseeable future ability. When website traffic boosts, you could increase extra means with just a few clicks or automatically using vehicle-scaling. When traffic drops, you can scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability resources. You are able to concentrate on developing your application in lieu of running infrastructure.
Containers are A different essential Device. A container packages your application and all the things it ought to run—code, libraries, settings—into a person device. This causes it to be straightforward to maneuver your app between environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application employs several containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If a person portion of your application crashes, it restarts it quickly.
Containers also ensure it is easy to individual elements of your application into companies. You are able to update or scale sections independently, which can be perfect for functionality and reliability.
Briefly, utilizing cloud and container instruments implies you could scale quickly, deploy easily, and Recuperate immediately when challenges take place. If you prefer your app to improve without boundaries, get started making use of these applications early. They conserve time, lower risk, and allow you to continue to be focused on creating, not correcting.
Monitor Every little thing
For those who don’t keep track of your application, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make far better selections as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking simple metrics like CPU utilization, memory, disk Room, and reaction time. These show you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you collect and visualize this information.
Don’t just check your servers—keep an eye on your application far too. Control how much time it's going to take for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening within your code.
Arrange alerts for vital issues. As an example, Should your response time goes above a Restrict or simply a assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently before buyers even detect.
Checking is additionally helpful when you make variations. When you deploy a whole new characteristic and see a spike in errors or slowdowns, you could roll it back again just before it leads to serious problems.
As your app grows, targeted visitors and facts boost. Without checking, you’ll skip indications of difficulties till it’s much too late. But with the best tools set up, you remain on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about being familiar with your technique and making sure it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large corporations. Even little applications need a robust Basis. By developing thoroughly, optimizing wisely, and utilizing the ideal equipment, you could Construct applications that grow easily without the need of breaking under pressure. Start off small, Imagine large, and Create good.