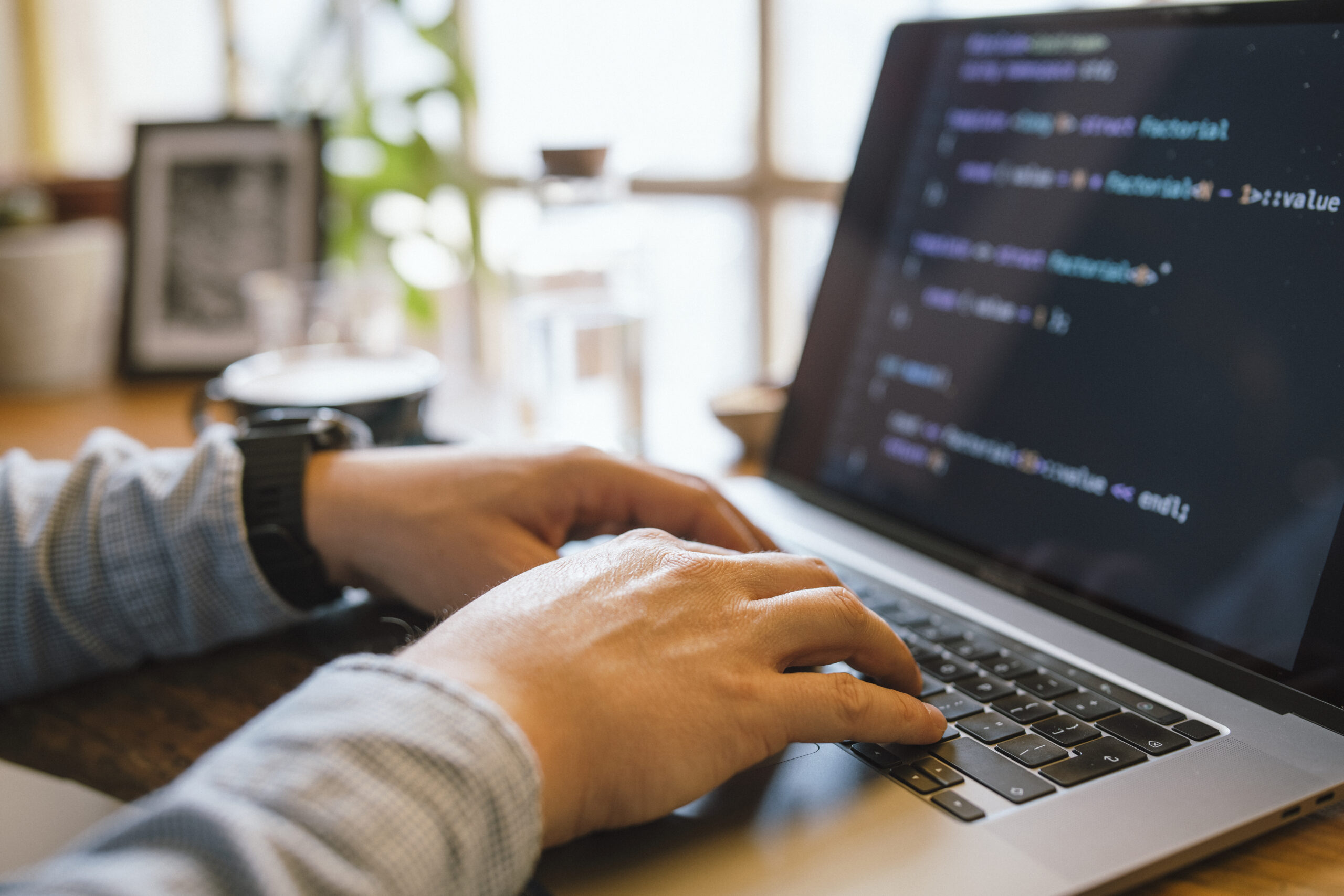
Debugging is Probably the most crucial — still normally ignored — capabilities in a very developer’s toolkit. It isn't really just about fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to resolve troubles successfully. Regardless of whether you are a rookie or a seasoned developer, sharpening your debugging capabilities can save hrs of disappointment and dramatically boost your efficiency. Here are many methods that can help builders degree up their debugging video game by me, Gustavo Woltmann.
Learn Your Resources
On the list of quickest ways builders can elevate their debugging skills is by mastering the tools they use daily. While creating code is a person Portion of growth, recognizing ways to interact with it efficiently for the duration of execution is equally essential. Modern day progress environments occur Geared up with powerful debugging abilities — but quite a few developers only scratch the area of what these instruments can do.
Take, for instance, an Built-in Growth Surroundings (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code to the fly. When utilized the right way, they Allow you to notice specifically how your code behaves during execution, that's a must have for tracking down elusive bugs.
Browser developer applications, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, view true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can switch frustrating UI concerns into workable tasks.
For backend or process-degree builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB provide deep control in excess of running procedures and memory administration. Understanding these tools could have a steeper Studying curve but pays off when debugging overall performance challenges, memory leaks, or segmentation faults.
Over and above your IDE or debugger, turn out to be snug with Edition Command methods like Git to grasp code record, come across the precise instant bugs were introduced, and isolate problematic modifications.
Eventually, mastering your equipment suggests likely outside of default configurations and shortcuts — it’s about acquiring an personal knowledge of your development atmosphere in order that when troubles occur, you’re not dropped at nighttime. The higher you already know your resources, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and sometimes disregarded — actions in efficient debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers require to create a dependable ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, correcting a bug gets a sport of opportunity, frequently leading to squandered time and fragile code improvements.
The initial step in reproducing a challenge is collecting as much context as you possibly can. Inquire concerns like: What steps brought about the issue? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've, the a lot easier it will become to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected more than enough details, try to recreate the situation in your local natural environment. This could signify inputting exactly the same facts, simulating comparable person interactions, or mimicking method states. If The problem appears intermittently, take into account writing automated assessments that replicate the edge situations or point out transitions concerned. These assessments not only support expose the condition but in addition reduce regressions Later on.
From time to time, the issue could possibly be ecosystem-particular — it would transpire only on certain working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these kinds of bugs.
Reproducing the challenge isn’t merely a phase — it’s a way of thinking. It calls for patience, observation, as well as a methodical tactic. But when you can persistently recreate the bug, you might be currently midway to correcting it. By using a reproducible scenario, You should utilize your debugging instruments extra correctly, exam likely fixes properly, and communicate far more clearly with your crew or buyers. It turns an summary grievance into a concrete challenge — and that’s the place builders prosper.
Study and Realize the Error Messages
Mistake messages are frequently the most worthy clues a developer has when a little something goes wrong. Instead of seeing them as aggravating interruptions, builders really should study to deal with error messages as immediate communications through the technique. They normally show you what precisely transpired, wherever it took place, and at times even why it happened — if you know the way to interpret them.
Start off by reading through the message diligently As well as in total. Numerous builders, particularly when under time tension, glance at the very first line and straight away start out producing assumptions. But further from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines — examine and realize them to start with.
Split the mistake down into areas. Is it a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line range? What module or perform activated it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically follow predictable designs, and Mastering to recognize these can dramatically increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals conditions, it’s essential to examine the context where the mistake occurred. Check out linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede much larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Finding out to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems more quickly, lessen debugging time, and turn into a far more successful and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging stages contain DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for in-depth diagnostic information during advancement, Information for general events (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual problems, and Lethal once the method can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on key gatherings, condition changes, enter/output values, and demanding decision factors inside your code.
Structure your log messages Obviously and continuously. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all devoid of halting the program. They’re Specially beneficial in generation environments exactly where stepping as a result of code isn’t achievable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, smart logging is about equilibrium and clarity. Having a properly-thought-out logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently identify and resolve bugs, developers ought to solution the process like a detective solving a thriller. This way of thinking allows stop working complex difficulties into workable pieces and comply with clues logically to uncover the foundation cause.
Commence by accumulating evidence. Consider the signs of the situation: mistake messages, incorrect output, or overall performance troubles. The same as a detective surveys against the law scene, collect as much appropriate information as you may with out jumping to conclusions. Use logs, take a look at conditions, and user studies to piece together a transparent photograph of what’s going on.
Up coming, variety hypotheses. Ask yourself: What could be causing this behavior? Have any changes recently been made into the codebase? Has this issue happened before below similar situations? The objective will be to slim down options and determine opportunity culprits.
Then, exam your theories systematically. Seek to recreate the condition in a controlled environment. For those who suspect a selected purpose or element, isolate it and validate if The difficulty persists. Just like a detective conducting interviews, inquire your code inquiries and Enable the effects direct you closer to the reality.
Shell out close notice to modest specifics. Bugs often disguise while in the least predicted locations—similar to a missing semicolon, an off-by-just one error, or simply a race problem. Be complete and individual, resisting the urge to patch The difficulty without having fully comprehension it. Temporary fixes may possibly hide the more info true trouble, only for it to resurface later on.
Last of all, hold notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical skills, strategy challenges methodically, and become simpler at uncovering hidden difficulties in complex techniques.
Publish Checks
Writing tests is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that provides you self confidence when building variations towards your codebase. A well-tested application is easier to debug because it allows you to pinpoint precisely in which and when a challenge happens.
Begin with unit exams, which give attention to personal functions or modules. These little, isolated tests can rapidly reveal whether or not a specific bit of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know the place to seem, substantially lowering the time spent debugging. Device assessments are Specifically helpful for catching regression bugs—problems that reappear after Beforehand staying mounted.
Subsequent, combine integration tests and conclusion-to-conclude exams into your workflow. These help make sure a variety of areas of your application get the job done collectively easily. They’re significantly handy for catching bugs that take place in complex units with a number of components or expert services interacting. If one thing breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing checks also forces you to think critically regarding your code. To test a element correctly, you would like to comprehend its inputs, envisioned outputs, and edge situations. This volume of comprehension naturally qualified prospects to higher code composition and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you can give attention to correcting the bug and watch your examination go when The difficulty is settled. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the discouraging guessing game into a structured and predictable approach—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to be immersed in the situation—gazing your monitor for hours, attempting Remedy soon after Option. But One of the more underrated debugging applications is simply stepping absent. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move about, extend, or do some thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to faster and simpler debugging Ultimately.
In brief, having breaks will not be a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing important in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring yourself a couple of crucial queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with far better techniques like device screening, code reviews, or logging? The answers frequently reveal blind spots in your workflow or being familiar with and assist you build stronger coding habits moving ahead.
Documenting bugs can even be an outstanding practice. Preserve a developer journal or maintain a log where you Take note down bugs you’ve encountered, the way you solved them, and Whatever you acquired. Eventually, you’ll begin to see designs—recurring troubles or prevalent faults—you can proactively prevent.
In staff environments, sharing Whatever you've realized from a bug with your friends might be Specifically potent. Whether it’s by way of a Slack message, a short publish-up, or A fast information-sharing session, assisting others steer clear of the identical problem boosts workforce effectiveness and cultivates a stronger Discovering lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as necessary parts of your enhancement journey. All things considered, a few of the finest developers are usually not those who write great code, but people that continuously study from their blunders.
Eventually, Each and every bug you repair adds a completely new layer to the skill set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, additional able developer as a result of it.
Summary
Enhancing your debugging capabilities takes time, follow, and tolerance — however the payoff is big. It can make you a more effective, self-confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.